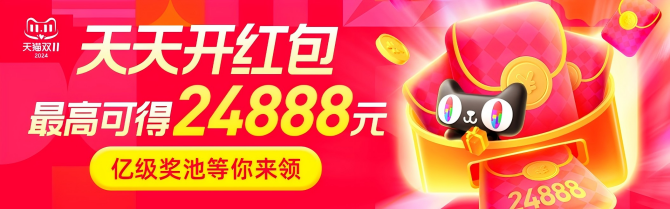
刚刚开始学习Java,对Java不是很熟悉,但是自己的兴趣挺喜欢Java。现在自己在自学Java做一个小游戏,坦克大战。
自己现在完成了画出自己的坦克和坦克的移动方向。希望各位大神指导一下我这个刚刚学Java的新手小白。
我会每完成一个阶段,就更新一下博客,跟进一下自己的学习进度。
在做这个坦克大战的时候,不知道从哪里开始做起,再来自己就觉得先画出自己的坦克吧。
下面是创建坦克类(方便以后能创建更加多的坦克),再创建自己的坦克继承坦克类。
package tankgames; import java.awt.*; //创建坦克类 public class Tank { int x,y,w,h; int speed; int direct; Color c1,c2,c3; public Tank(){ x=230; y=300; w=5; h=60; speed=10; direct=0; } public Tank(int x, int y, int w, int h, int speed, int direct) { super(); this.x = x; this.y = y; this.w = w; this.h = h; this.speed = speed; this.direct = direct; } } //创建我的坦克子类 class MyTank extends Tank{ //创建颜色对象,并设置颜色 public MyTank(){ this.c1 = new Color(128,128,128); this.c2 = new Color(0,255,0); this.c3 = new Color(0,0,255); } public MyTank(int x, int y, int w, int h, int speed, int direct) { super(x, y, w, h, speed, direct); this.c1 = new Color(128,128,128); this.c2 = new Color(0,255,0); this.c3 = new Color(0,0,255); } }
创建完自己的坦克,要写个主函数来画出自己的坦克,以下是画出自己的坦克:
package tankgames; import java.awt.*; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.*; public class TankGame extends JFrame{ private static final long serialVersionUID = 1L; MyPanel mp; public static void main(String[] args) { new TankGame().setVisible(true); } public TankGame(){ this.setTitle("坦克大战"); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setResizable(false); this.setBounds(100, 100, 600, 500); //创建我的面板对象,并添加到我的窗体里面 mp = new MyPanel(); this.getContentPane().add(mp); //注册键盘事件监听 this.addKeyListener(mp); } } //创建我的面板子类 class MyPanel extends JPanel implements KeyListener{ MyTank myTank; private static final long serialVersionUID = 1L; public MyPanel(){ this.setLayout(null); myTank = new MyTank(); } //面板容器的设置和组件在面板容器里显示 public void paint(Graphics g){ super.paint(g); g.setColor(new Color(255,255,221)); g.fill3DRect(0, 0, 500, 400, false); drawTank(myTank,g); } //画坦克 public void drawTank(Tank t,Graphics g){ int x = t.x, y = t.y, w = t.w, h = t.h; //画出向上的坦克 if(t.direct == 0){ Graphics2D g2d = (Graphics2D)g; g.setColor(t.c1); g.fill3DRect(x, y, w, h, false); g.fill3DRect(x+7*w, y, w, h, false); g.setColor(t.c2); g.fill3DRect(x+w, y+2*w, 6*w, 8*w, false); g.fillOval(x+2*w, y+4*w, 4*w, 4*w); g2d.setColor(t.c3); g2d.setStroke(new BasicStroke(5.0f)); g2d.drawLine(x+4*w, y, x+4*w, y+6*w); } //画出向下的坦克 else if(t.direct == 2){ Graphics2D g2d = (Graphics2D)g; g.setColor(t.c1); g.fill3DRect(x, y, w, h, false); g.fill3DRect(x+7*w, y, w, h, false); g.setColor(t.c2); g.fill3DRect(x+w, y+2*w, 6*w, 8*w, false); g.fillOval(x+2*w, y+4*w, 4*w, 4*w); g2d.setColor(t.c3); g2d.setStroke(new BasicStroke(5.0f)); g2d.drawLine(x+4*w, y+6*w, x+4*w, y+12*w); } //画出向左的坦克 else if(t.direct == 3){ Graphics2D g2d = (Graphics2D)g; g.setColor(t.c1); g.fill3DRect(x, y, h, w, false); g.fill3DRect(x, y+7*w, h, w, false); g.setColor(t.c2); g.fill3DRect(x+2*w, y+w, 8*w, 6*w, false); g.fillOval(x+4*w, y+2*w, 4*w, 4*w); g2d.setColor(t.c3); g2d.setStroke(new BasicStroke(5.0f)); g2d.drawLine(x, y+4*w, x+6*w, y+4*w); } //画出向右的坦克 else if(t.direct ==1){ Graphics2D g2d = (Graphics2D)g; g.setColor(t.c1); g.fill3DRect(x, y, h, w, false); g.fill3DRect(x, y+7*w, h, w, false); g.setColor(t.c2); g.fill3DRect(x+2*w, y+w, 8*w, 6*w, false); g.fillOval(x+4*w, y+2*w, 4*w, 4*w); g2d.setColor(t.c3); g2d.setStroke(new BasicStroke(5.0f)); g2d.drawLine(x+6*w, y+4*w, x+12*w, y+4*w); } } @Override public void keyTyped(KeyEvent e) { } //我的坦克移动 @Override public void keyPressed(KeyEvent e) { //向左移动 if(e.getKeyCode() == KeyEvent.VK_LEFT || e.getKeyCode() == KeyEvent.VK_A){ myTank.x -= myTank.speed; myTank.direct = 3; } //向右移动 else if(e.getKeyCode() == KeyEvent.VK_RIGHT || e.getKeyCode() == KeyEvent.VK_D){ myTank.x += myTank.speed; myTank.direct = 1; } //向上移动 else if(e.getKeyCode() == KeyEvent.VK_UP || e.getKeyCode() == KeyEvent.VK_W){ myTank.y -= myTank.speed; myTank.direct =0; } //向下移动 else if(e.getKeyCode() == KeyEvent.VK_DOWN || e.getKeyCode() == KeyEvent.VK_S){ myTank.y += myTank.speed; myTank.direct = 2; } //关闭游戏 else if(e.getKeyCode() == KeyEvent.VK_ESCAPE){ System.exit(0); } //刷新图形 this.repaint(); } @Override public void keyReleased(KeyEvent e) { } }
我画自己的坦克只是计算好位置,画出图形。
再来自己到最后,有一个疑惑,自己不懂。
坦克每次改变方向移动时,能不能有更加方便的方法来封装上面画图形时自己手动改变位置?若画其他坦克,是不是还要自己手动改变坦克的位置?这里自己觉得处理不是很好,现在还在想怎么能更加完美,更加方便。
最新评论