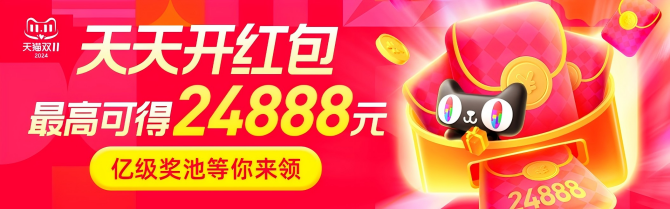
#include <iostream> // typeid testing ////////////////////////////////////////////////////////// int main() { { int v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { long v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { float v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { double v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { char v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { uint8_t v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { uint16_t v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { uint32_t v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } { uint64_t v(0); std::cout << typeid(v).name() << std::endl; std::cout << typeid(v).raw_name() << std::endl; } return 0; }
使用示例
#include <iostream> // typeid testing ////////////////////////////////////////////////////////// int main() { int a(0); // 错误调用!!!!!! // 比较两个字符串不能直接使用 == // 这是两个 cosnt char * 类型的变量,==执行的是地址的比较。所以会返回false!!!! if (typeid(a).name() == "int") { // do something std::cout << "It is integer. by name" << std::endl; } // 使用string来比较ok if (std::string(typeid(a).name()) == "int") { // do something std::cout << "It is integer. if (std::string(typeid(a).name()) == "int")" << std::endl; } // 这样也可以,返回的是同一个对象,字符串的地址自然也一样。 auto p1 = typeid(a).name(); auto p2 = typeid(1).name(); if (typeid(a) == typeid(1)) // 比较对象 { // do something std::cout << "It is integer. if (typeid(a) == typeid(1))" << std::endl; } if (typeid(a) == typeid(1.0f)) // 比较对象 { // do something std::cout << "It is integer. if (typeid(a) == typeid(1.0f))" << std::endl; } if (typeid(a) == typeid(int(1.0))) // 比较对象 { // do something std::cout << "It is integer. if (typeid(a) == typeid(int(1.0)))" << std::endl; } return 0; }
最新评论